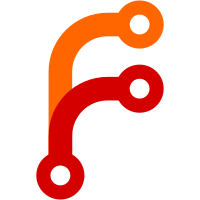
* Update schema * Update queries, mutations, and types * Add render with dividers util function * Add plugin details channels card component * Update plugin details to use channels * Update stories * Update plugin configuration type across the app, fix some other types, temporarily comment some things out in plugins list so types match" * Update schema * Update types * Update plugins list to show channels and global statuses, add plugin channel status, update status label component * Add render with dividers util function * Comment out some stuff for types to match - temporary * Add useChannelsSearchWithLoadMore util to imitate loading more from backend for channels list with load more * Change filters logic to be able to display multiple fields in a field section and add it to plugins view * Add scroll option to plugin availability popup on plugin list * Fix plugin list page story * Temporarily comment some stuff out, fix some types * Add filters errors WIP * Fix filters not updating list * Add error handling to plugins list filters and filters in general * Rename some components according to review * Move useChannelsSearch and useChannelsSearchWithLoadMore to hooks, change some imports accordingly * Fix imports * Move render collection with dividers to a component, fix usages * Replace channels with load more and search query to base channels query * Change render with dividers function to take in a component instead of render function * Update tests * Extract messages * Remove unnecessary imports * Fix filters - autocomplete messing items order sometimes & some fields not working * Update plugin update mutation variables - change channelId to channel * fix failing tests * Add test ids * fix failing tests * fix failing tests * Rename misc.tsx to ts * Remove usage of render collection with diviers, change it to CollectionWithDividers component * Remove unnecessary imports * Update messages ids * Update snapshots Co-authored-by: Karolina Rakoczy <rakoczy.karolina@gmail.com>
128 lines
4.5 KiB
TypeScript
128 lines
4.5 KiB
TypeScript
import { ConfigurationTypeFieldEnum } from "@saleor/types/globalTypes";
|
|
|
|
import { Plugin_plugin } from "./types/Plugin";
|
|
import { Plugins_plugins_edges_node } from "./types/Plugins";
|
|
|
|
export const pluginList: Plugins_plugins_edges_node[] = [
|
|
{
|
|
__typename: "Plugin",
|
|
globalConfiguration: null,
|
|
channelConfigurations: [
|
|
{
|
|
__typename: "PluginConfiguration",
|
|
active: true,
|
|
configuration: [],
|
|
channel: {
|
|
__typename: "Channel",
|
|
id: "channel-1",
|
|
name: "channel 1",
|
|
slug: "channel-1"
|
|
}
|
|
}
|
|
],
|
|
description:
|
|
"Lorem ipsum dolor sit amet enim. Etiam ullamcorper. Suspendisse a pellentesque dui, non felis. Maecenas malesuada elit lectus felis, malesuada ultricies. Curabitur et ligula. Ut molestie a, ultricies porta urna. Vestibulum commodo volutpat a, convallis ac, laoreet enim. Phasellus fermentum in, dolor. Pellentesque facilisis. Nulla imperdiet sit amet magna.",
|
|
id: "Jzx123sEt==",
|
|
name: "Avalara"
|
|
},
|
|
{
|
|
__typename: "Plugin",
|
|
globalConfiguration: null,
|
|
channelConfigurations: [
|
|
{
|
|
__typename: "PluginConfiguration",
|
|
active: true,
|
|
configuration: [],
|
|
channel: {
|
|
__typename: "Channel",
|
|
id: "channel-1",
|
|
name: "channel 1",
|
|
slug: "channel-1"
|
|
}
|
|
}
|
|
],
|
|
description:
|
|
"Lorem ipsum dolor sit amet enim. Etiam ullamcorper. Suspendisse a pellentesque dui, non felis. Maecenas malesuada elit lectus felis, malesuada ultricies. Curabitur et ligula. Ut molestie a, ultricies porta urna. Vestibulum commodo volutpat a, convallis ac, laoreet enim. Phasellus fermentum in, dolor. Pellentesque facilisis. Nulla imperdiet sit amet magna.",
|
|
id: "Jzx123sEt==",
|
|
name: "VatLayer"
|
|
}
|
|
];
|
|
export const plugin: Plugin_plugin = {
|
|
__typename: "Plugin",
|
|
globalConfiguration: null,
|
|
channelConfigurations: [
|
|
{
|
|
__typename: "PluginConfiguration",
|
|
active: true,
|
|
channel: {
|
|
__typename: "Channel",
|
|
id: "channel-1",
|
|
name: "channel 1",
|
|
slug: "channel-1"
|
|
},
|
|
configuration: [
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "Provide user or account details",
|
|
label: "Username or account",
|
|
name: "Username or account",
|
|
type: ConfigurationTypeFieldEnum.STRING,
|
|
value: "avatax_user"
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "Provide password or license details",
|
|
label: "Password or license",
|
|
name: "Password or license",
|
|
type: ConfigurationTypeFieldEnum.STRING,
|
|
value: "TEM8S2-2ET83-CGKP1-DPSI2-EPZO1"
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "This key will enable you to connect to Avatax API",
|
|
label: "API key",
|
|
name: "apiKey",
|
|
type: ConfigurationTypeFieldEnum.SECRET,
|
|
value: "9ab9"
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "",
|
|
label: "Password",
|
|
name: "password",
|
|
type: ConfigurationTypeFieldEnum.PASSWORD,
|
|
value: ""
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "",
|
|
label: "Empty Password",
|
|
name: "password-not-set",
|
|
type: ConfigurationTypeFieldEnum.PASSWORD,
|
|
value: null
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "Determines if Saleor should use Avatax sandbox API.",
|
|
label: "Use sandbox",
|
|
name: "Use sandbox",
|
|
type: ConfigurationTypeFieldEnum.BOOLEAN,
|
|
value: "true"
|
|
},
|
|
{
|
|
__typename: "ConfigurationItem",
|
|
helpText: "This is a multiline field",
|
|
label: "Multiline Field",
|
|
name: "multiline-field",
|
|
type: ConfigurationTypeFieldEnum.MULTILINE,
|
|
value:
|
|
"Lorem ipsum\ndolor sit\namet enim.\nEtiam ullamcorper.\nSuspendisse a\npellentesque dui,\nnon felis."
|
|
}
|
|
]
|
|
}
|
|
],
|
|
description:
|
|
"Lorem ipsum dolor sit amet enim. Etiam ullamcorper. Suspendisse a pellentesque dui, non felis. Maecenas malesuada elit lectus felis, malesuada ultricies. Curabitur et ligula. Ut molestie a, ultricies porta urna. Vestibulum commodo volutpat a, convallis ac, laoreet enim. Phasellus fermentum in, dolor. Pellentesque facilisis. Nulla imperdiet sit amet magna.",
|
|
id: "UGx1Z2luQ29uZmlndXJhdGlvbjoy",
|
|
name: "Username or account"
|
|
};
|