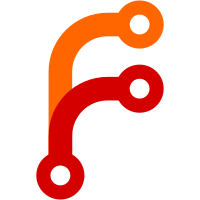
* Create user addresses select modal * Prepare user addresses select modal form * Add user addresses select modal to order draft details page * Update draft order validation of adresses in edit modal * Add Customer Change modal * Update snapshots and messages * Indication of address form errors by address type * Refactor addresses submiting * Refactor address transform functions * Add data-testids to addresses change dialog * Update customer address choice style * Trigger CI * Update customer addresses edit flow * Move styles outside of component files * Refactor after review * Refactor after review * Do not update customer if the same selected * Handle setting adress after edit customer with no addresses * Trigger CI
51 lines
1.4 KiB
TypeScript
51 lines
1.4 KiB
TypeScript
import { AddressTypeInput } from "@saleor/customers/types";
|
|
import { AccountErrorFragment } from "@saleor/fragments/types/AccountErrorFragment";
|
|
import { transformFormToAddressInput } from "@saleor/misc";
|
|
import {
|
|
AccountErrorCode,
|
|
AddressInput,
|
|
AddressTypeEnum
|
|
} from "@saleor/types/globalTypes";
|
|
import { add, remove } from "@saleor/utils/lists";
|
|
import { useState } from "react";
|
|
|
|
interface UseAddressValidation<TInput, TOutput> {
|
|
errors: AccountErrorFragment[];
|
|
submit: (data: TInput & AddressTypeInput) => TOutput;
|
|
}
|
|
|
|
function useAddressValidation<TInput, TOutput>(
|
|
onSubmit: (address: TInput & AddressInput) => TOutput,
|
|
addressType?: AddressTypeEnum
|
|
): UseAddressValidation<TInput, TOutput> {
|
|
const [validationErrors, setValidationErrors] = useState<
|
|
AccountErrorFragment[]
|
|
>([]);
|
|
|
|
const countryRequiredError: AccountErrorFragment = {
|
|
__typename: "AccountError",
|
|
code: AccountErrorCode.REQUIRED,
|
|
field: "country",
|
|
addressType
|
|
};
|
|
|
|
return {
|
|
errors: validationErrors,
|
|
submit: (data: TInput & AddressTypeInput) => {
|
|
try {
|
|
setValidationErrors(
|
|
remove(
|
|
countryRequiredError,
|
|
validationErrors,
|
|
(a, b) => a.field === b.field
|
|
)
|
|
);
|
|
return onSubmit(transformFormToAddressInput(data));
|
|
} catch {
|
|
setValidationErrors(add(countryRequiredError, validationErrors));
|
|
}
|
|
}
|
|
};
|
|
}
|
|
|
|
export default useAddressValidation;
|