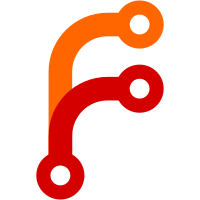
* Add new Apps List * Update apps routing * Add marketplace apps list * Update apps urls * Update app list style * Add installed apps section * Update apps sections and connect actions with mutations * Add latest missing buttons and labels to installed apps list * Update installed apps list * Update installed apps list * Add tests and marketplace error handling * Update environment configuration * Update GitHub actions env configuration * Refactor AppListCard component * Test InstallWithManifestFormButton * Test AppListCard * Extract InstalledAppListRow with tests * Update GitHub actions env configuration * Tests of apps dialogs * Update GitHub actions env configuration * Update messages * Update GitHub actions env configuration * Quote untrusted GitHub actions variables * Change useFetch to useMarketplaceApps and add tests * Fix strict null check errors * Refactor apps details components * Add strict null checks for /new-apps/ components
76 lines
1 KiB
TypeScript
76 lines
1 KiB
TypeScript
import { gql } from "@apollo/client";
|
|
|
|
export const appManifestFragment = gql`
|
|
fragment AppManifest on Manifest {
|
|
identifier
|
|
version
|
|
about
|
|
name
|
|
appUrl
|
|
configurationUrl
|
|
tokenTargetUrl
|
|
dataPrivacy
|
|
dataPrivacyUrl
|
|
homepageUrl
|
|
supportUrl
|
|
permissions {
|
|
code
|
|
name
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const appFragment = gql`
|
|
fragment App on App {
|
|
id
|
|
name
|
|
created
|
|
isActive
|
|
type
|
|
homepageUrl
|
|
appUrl
|
|
manifestUrl
|
|
configurationUrl
|
|
supportUrl
|
|
version
|
|
accessToken
|
|
privateMetadata {
|
|
key
|
|
value
|
|
}
|
|
metadata {
|
|
key
|
|
value
|
|
}
|
|
tokens {
|
|
authToken
|
|
id
|
|
name
|
|
}
|
|
webhooks {
|
|
...Webhook
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const appListItemFragment = gql`
|
|
fragment AppListItem on App {
|
|
id
|
|
name
|
|
isActive
|
|
type
|
|
appUrl
|
|
manifestUrl
|
|
version
|
|
permissions {
|
|
...AppPermission
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const appPermissionFragment = gql`
|
|
fragment AppPermission on Permission {
|
|
name
|
|
code
|
|
}
|
|
`;
|