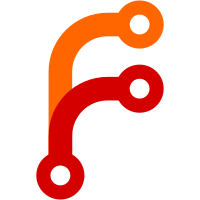
* Minor fixes for intl messages * Add esbuild-loader * switch from babel to esbuild-loader * use formatjs enforce-id linter * Generate ids for intl messages * id format defined by idInterpolationPattern * Modify intl messages extraction * remove react-intl-translations-manager * remove transpile-tx.js * use formatjs cli * Modify defaultMessages.json * modify ids in defaultMessages.json with defined idInterpolationPattern * Fix errors * Fix page crash * Use babel to transpile tests * Fix useStateFromProps * Improve render count * Add test to useStateFromProps * Fix reloading state buh * Do not check if form with channels is dirty * Stop blocking save if form has not changed * Remove debug code * Fix form disabling * Fix variant selection checkbox onClick * Update translations * Update messages * Use esbuild to build storybook Co-authored-by: Bartłomiej Wiaduch <tukan2can@gmail.com> Co-authored-by: Jakub Majorek <majorek.jakub@gmail.com>
109 lines
2.7 KiB
TypeScript
109 lines
2.7 KiB
TypeScript
import {
|
|
FormControl,
|
|
FormControlLabel,
|
|
FormHelperText,
|
|
MenuItem,
|
|
Radio,
|
|
RadioGroup
|
|
} from "@material-ui/core";
|
|
import classNames from "classnames";
|
|
import React from "react";
|
|
import { FormattedMessage } from "react-intl";
|
|
|
|
import { useStyles } from "./styles";
|
|
|
|
export interface RadioGroupFieldChoice<
|
|
T extends string | number = string | number
|
|
> {
|
|
disabled?: boolean;
|
|
value: T;
|
|
label: React.ReactNode;
|
|
}
|
|
|
|
interface RadioGroupFieldProps {
|
|
alignTop?: boolean;
|
|
choices: RadioGroupFieldChoice[];
|
|
className?: string;
|
|
innerContainerClassName?: string;
|
|
disabled?: boolean;
|
|
error?: boolean;
|
|
hint?: string;
|
|
label?: React.ReactNode;
|
|
name?: string;
|
|
value: string | number;
|
|
variant?: "block" | "inline" | "inlineJustify";
|
|
onChange: (event: React.ChangeEvent<any>) => void;
|
|
}
|
|
|
|
export const RadioGroupField: React.FC<RadioGroupFieldProps> = props => {
|
|
const {
|
|
alignTop,
|
|
className,
|
|
disabled,
|
|
error,
|
|
label,
|
|
choices,
|
|
value,
|
|
onChange,
|
|
name,
|
|
hint,
|
|
variant = "block",
|
|
innerContainerClassName
|
|
} = props;
|
|
const classes = useStyles(props);
|
|
|
|
return (
|
|
<FormControl
|
|
className={classNames(classes.root, className, {
|
|
[classes.rootNoLabel]: !label
|
|
})}
|
|
error={error}
|
|
disabled={disabled}
|
|
>
|
|
{!!label && <label className={classes.formLabel}>{label}</label>}
|
|
<RadioGroup
|
|
aria-label={name}
|
|
name={name}
|
|
value={value}
|
|
onChange={onChange}
|
|
className={classNames({
|
|
[classes.radioGroupInline]: variant === "inline",
|
|
[innerContainerClassName]: !!innerContainerClassName
|
|
})}
|
|
>
|
|
{choices.length > 0 ? (
|
|
choices.map(choice => (
|
|
<FormControlLabel
|
|
disabled={choice.disabled}
|
|
value={choice.value}
|
|
className={classNames({
|
|
[classes.radioLabel]: variant !== "inline",
|
|
[classes.radioLabelInline]: variant === "inline"
|
|
})}
|
|
classes={{
|
|
label: classes.label
|
|
}}
|
|
control={
|
|
<Radio
|
|
className={classNames({
|
|
[classes.alignTop]: alignTop
|
|
})}
|
|
color="primary"
|
|
/>
|
|
}
|
|
label={choice.label}
|
|
key={choice.value}
|
|
/>
|
|
))
|
|
) : (
|
|
<MenuItem disabled={true}>
|
|
<FormattedMessage id="hX5PAb" defaultMessage="No results found" />
|
|
</MenuItem>
|
|
)}
|
|
</RadioGroup>
|
|
{hint && <FormHelperText>{hint}</FormHelperText>}
|
|
</FormControl>
|
|
);
|
|
};
|
|
RadioGroupField.displayName = "RadioGroupField";
|
|
export default RadioGroupField;
|