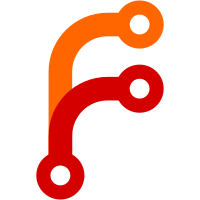
* Use generated hooks in apps * Remove unused files * Use proper types in apps * Use generated hooks in attributes * Use generated hooks in auth module * Use generated hooks in categories * Use generated hooks in channels * Use generated types in collections * Remove legacy types from background tasks * Use generated hooks in customers * Use generated hooks in discounts * Use generated hook in file upload * Use generated types in gift cards * Use generated types in home * Use generated hooks in navigation * Use generated hooks in orders * Use generated hooks in pages * Use generated hooks in page types * Use generated hooks in permission groups * Use generated hooks in plugins * Use generated hooks in products * Use fragment to mark product variants * Improve code a bit * Use generated hooks in page types * Use generated types in searches * Use generated hooks in shipping * Use generated hooks in site settings * Use generated hooks in staff members * Use generated hooks in taxes * Place all gql generated files in one directory * Use generated hooks in translations * Use global types from new generated module * Use generated hooks in warehouses * Use generated hooks in webhooks * Use generated fragment types * Unclutter types * Remove hoc components * Split hooks and types * Fetch introspection file * Delete obsolete schema file * Fix rebase artifacts * Fix autoreplace * Fix auth provider tests * Fix urls * Remove leftover types * Fix rebase artifacts
263 lines
5.4 KiB
TypeScript
263 lines
5.4 KiB
TypeScript
import { gql } from "@apollo/client";
|
|
|
|
export const updateProductTranslations = gql`
|
|
mutation UpdateProductTranslations(
|
|
$id: ID!
|
|
$input: TranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
productTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...ProductTranslateErrorFragment
|
|
}
|
|
product {
|
|
id
|
|
name
|
|
description
|
|
seoDescription
|
|
seoTitle
|
|
translation(languageCode: $language) {
|
|
id
|
|
description
|
|
language {
|
|
code
|
|
language
|
|
}
|
|
name
|
|
seoDescription
|
|
seoTitle
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateProductVariantTranslations = gql`
|
|
mutation UpdateProductVariantTranslations(
|
|
$id: ID!
|
|
$input: NameTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
productVariantTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...ProductVariantTranslateErrorFragment
|
|
}
|
|
productVariant {
|
|
id
|
|
name
|
|
translation(languageCode: $language) {
|
|
id
|
|
name
|
|
language {
|
|
code
|
|
language
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateCategoryTranslations = gql`
|
|
mutation UpdateCategoryTranslations(
|
|
$id: ID!
|
|
$input: TranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
categoryTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...CategoryTranslateErrorFragment
|
|
}
|
|
category {
|
|
id
|
|
name
|
|
description
|
|
seoDescription
|
|
seoTitle
|
|
translation(languageCode: $language) {
|
|
id
|
|
description
|
|
language {
|
|
language
|
|
}
|
|
name
|
|
seoDescription
|
|
seoTitle
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateCollectionTranslations = gql`
|
|
mutation UpdateCollectionTranslations(
|
|
$id: ID!
|
|
$input: TranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
collectionTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...CollectionTranslateErrorFragment
|
|
}
|
|
collection {
|
|
id
|
|
name
|
|
description
|
|
seoDescription
|
|
seoTitle
|
|
translation(languageCode: $language) {
|
|
id
|
|
description
|
|
language {
|
|
language
|
|
}
|
|
name
|
|
seoDescription
|
|
seoTitle
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updatePageTranslations = gql`
|
|
mutation UpdatePageTranslations(
|
|
$id: ID!
|
|
$input: PageTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
pageTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...PageTranslateErrorFragment
|
|
}
|
|
page {
|
|
...PageTranslation
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateVoucherTranslations = gql`
|
|
mutation UpdateVoucherTranslations(
|
|
$id: ID!
|
|
$input: NameTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
voucherTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...VoucherTranslateErrorFragment
|
|
}
|
|
voucher {
|
|
id
|
|
name
|
|
translation(languageCode: $language) {
|
|
id
|
|
language {
|
|
code
|
|
language
|
|
}
|
|
name
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateSaleTranslations = gql`
|
|
mutation UpdateSaleTranslations(
|
|
$id: ID!
|
|
$input: NameTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
saleTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...SaleTranslateErrorFragment
|
|
}
|
|
sale {
|
|
id
|
|
name
|
|
translation(languageCode: $language) {
|
|
id
|
|
language {
|
|
code
|
|
language
|
|
}
|
|
name
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateAttributeTranslations = gql`
|
|
mutation UpdateAttributeTranslations(
|
|
$id: ID!
|
|
$input: NameTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
attributeTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...AttributeTranslateErrorFragment
|
|
}
|
|
attribute {
|
|
id
|
|
name
|
|
translation(languageCode: $language) {
|
|
id
|
|
name
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateAttributeValueTranslations = gql`
|
|
mutation UpdateAttributeValueTranslations(
|
|
$id: ID!
|
|
$input: AttributeValueTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
attributeValueTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...AttributeValueTranslateErrorFragment
|
|
}
|
|
attributeValue {
|
|
id
|
|
name
|
|
richText
|
|
translation(languageCode: $language) {
|
|
id
|
|
name
|
|
richText
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const updateShippingMethodTranslations = gql`
|
|
mutation UpdateShippingMethodTranslations(
|
|
$id: ID!
|
|
$input: ShippingPriceTranslationInput!
|
|
$language: LanguageCodeEnum!
|
|
) {
|
|
shippingPriceTranslate(id: $id, input: $input, languageCode: $language) {
|
|
errors {
|
|
...ShippingPriceTranslateErrorFragment
|
|
}
|
|
shippingMethod {
|
|
id
|
|
name
|
|
description
|
|
translation(languageCode: $language) {
|
|
id
|
|
language {
|
|
language
|
|
}
|
|
name
|
|
description
|
|
}
|
|
}
|
|
}
|
|
}
|
|
`;
|