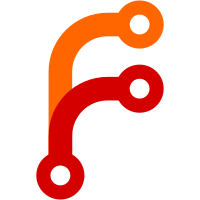
* Use generated hooks in apps * Remove unused files * Use proper types in apps * Use generated hooks in attributes * Use generated hooks in auth module * Use generated hooks in categories * Use generated hooks in channels * Use generated types in collections * Remove legacy types from background tasks * Use generated hooks in customers * Use generated hooks in discounts * Use generated hook in file upload * Use generated types in gift cards * Use generated types in home * Use generated hooks in navigation * Use generated hooks in orders * Use generated hooks in pages * Use generated hooks in page types * Use generated hooks in permission groups * Use generated hooks in plugins * Use generated hooks in products * Use fragment to mark product variants * Improve code a bit * Use generated hooks in page types * Use generated types in searches * Use generated hooks in shipping * Use generated hooks in site settings * Use generated hooks in staff members * Use generated hooks in taxes * Place all gql generated files in one directory * Use generated hooks in translations * Use global types from new generated module * Use generated hooks in warehouses * Use generated hooks in webhooks * Use generated fragment types * Unclutter types * Remove hoc components * Split hooks and types * Fetch introspection file * Delete obsolete schema file * Fix rebase artifacts * Fix autoreplace * Fix auth provider tests * Fix urls * Remove leftover types * Fix rebase artifacts
57 lines
1.6 KiB
TypeScript
57 lines
1.6 KiB
TypeScript
import { AddressTypeInput } from "@saleor/customers/types";
|
|
import {
|
|
AccountErrorCode,
|
|
AccountErrorFragment,
|
|
AddressInput,
|
|
AddressTypeEnum
|
|
} from "@saleor/graphql";
|
|
import { transformFormToAddressInput } from "@saleor/misc";
|
|
import { add, remove } from "@saleor/utils/lists";
|
|
import { useState } from "react";
|
|
|
|
interface UseAddressValidation<TInput, TOutput> {
|
|
errors: AccountErrorFragment[];
|
|
submit: (
|
|
data: TInput & AddressTypeInput
|
|
) => TOutput | Promise<AccountErrorFragment[]>;
|
|
}
|
|
|
|
function useAddressValidation<TInput, TOutput>(
|
|
onSubmit: (address: TInput & AddressInput) => TOutput,
|
|
addressType?: AddressTypeEnum
|
|
): UseAddressValidation<TInput, TOutput> {
|
|
const [validationErrors, setValidationErrors] = useState<
|
|
AccountErrorFragment[]
|
|
>([]);
|
|
|
|
const countryRequiredError: AccountErrorFragment = {
|
|
__typename: "AccountError",
|
|
code: AccountErrorCode.REQUIRED,
|
|
field: "country",
|
|
addressType,
|
|
message: "Country required"
|
|
};
|
|
|
|
return {
|
|
errors: validationErrors,
|
|
submit: (data: TInput & AddressTypeInput) => {
|
|
try {
|
|
setValidationErrors(
|
|
remove(
|
|
countryRequiredError,
|
|
validationErrors,
|
|
(a, b) => a.field === b.field
|
|
)
|
|
);
|
|
return onSubmit(transformFormToAddressInput(data));
|
|
} catch {
|
|
const errors = add(countryRequiredError, validationErrors);
|
|
setValidationErrors(errors);
|
|
// since every onSubmit must return Promise<error>
|
|
return Promise.resolve(errors);
|
|
}
|
|
}
|
|
};
|
|
}
|
|
|
|
export default useAddressValidation;
|