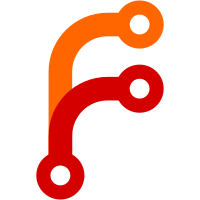
* Use generated hooks in apps * Remove unused files * Use proper types in apps * Use generated hooks in attributes * Use generated hooks in auth module * Use generated hooks in categories * Use generated hooks in channels * Use generated types in collections * Remove legacy types from background tasks * Use generated hooks in customers * Use generated hooks in discounts * Use generated hook in file upload * Use generated types in gift cards * Use generated types in home * Use generated hooks in navigation * Use generated hooks in orders * Use generated hooks in pages * Use generated hooks in page types * Use generated hooks in permission groups * Use generated hooks in plugins * Use generated hooks in products * Use fragment to mark product variants * Improve code a bit * Use generated hooks in page types * Use generated types in searches * Use generated hooks in shipping * Use generated hooks in site settings * Use generated hooks in staff members * Use generated hooks in taxes * Place all gql generated files in one directory * Use generated hooks in translations * Use global types from new generated module * Use generated hooks in warehouses * Use generated hooks in webhooks * Use generated fragment types * Unclutter types * Remove hoc components * Split hooks and types * Fetch introspection file * Delete obsolete schema file * Fix rebase artifacts * Fix autoreplace * Fix auth provider tests * Fix urls * Remove leftover types * Fix rebase artifacts
102 lines
3 KiB
TypeScript
102 lines
3 KiB
TypeScript
import { Card, CardContent, Typography } from "@material-ui/core";
|
|
import CardTitle from "@saleor/components/CardTitle";
|
|
import RadioGroupField from "@saleor/components/RadioGroupField";
|
|
import { AttributeTypeEnum } from "@saleor/graphql";
|
|
import { makeStyles } from "@saleor/macaw-ui";
|
|
import React from "react";
|
|
import { defineMessages, FormattedMessage, useIntl } from "react-intl";
|
|
|
|
import { AttributePageFormData } from "../AttributePage";
|
|
|
|
export interface AttributeOrganizationProps {
|
|
canChangeType: boolean;
|
|
data: AttributePageFormData;
|
|
disabled: boolean;
|
|
onChange: (event: React.ChangeEvent<any>) => void;
|
|
}
|
|
|
|
const messages = defineMessages({
|
|
contentAttribute: {
|
|
defaultMessage: "Content Attribute",
|
|
description: "attribute type"
|
|
},
|
|
productAttribute: {
|
|
defaultMessage: "Product Attribute",
|
|
description: "attribute type"
|
|
}
|
|
});
|
|
|
|
const useStyles = makeStyles(
|
|
theme => ({
|
|
card: {
|
|
overflow: "visible"
|
|
},
|
|
cardSubtitle: {
|
|
fontSize: theme.typography.body1.fontSize,
|
|
marginBottom: theme.spacing(0.5)
|
|
},
|
|
label: {
|
|
marginBottom: theme.spacing(0.5)
|
|
}
|
|
}),
|
|
{ name: "AttributeOrganization" }
|
|
);
|
|
|
|
const AttributeOrganization: React.FC<AttributeOrganizationProps> = props => {
|
|
const { canChangeType, data, disabled, onChange } = props;
|
|
|
|
const classes = useStyles(props);
|
|
const intl = useIntl();
|
|
|
|
return (
|
|
<Card>
|
|
<CardTitle
|
|
title={intl.formatMessage({
|
|
defaultMessage: "Organization",
|
|
description: "section header"
|
|
})}
|
|
/>
|
|
<CardContent>
|
|
{canChangeType ? (
|
|
<RadioGroupField
|
|
choices={[
|
|
{
|
|
label: intl.formatMessage(messages.productAttribute),
|
|
value: AttributeTypeEnum.PRODUCT_TYPE
|
|
},
|
|
{
|
|
label: intl.formatMessage(messages.contentAttribute),
|
|
value: AttributeTypeEnum.PAGE_TYPE
|
|
}
|
|
]}
|
|
disabled={disabled}
|
|
label={
|
|
<>
|
|
<FormattedMessage defaultMessage="Attribute Class" />
|
|
<Typography variant="caption">
|
|
<FormattedMessage defaultMessage="Define where this attribute should be used in Saleor system" />
|
|
</Typography>
|
|
</>
|
|
}
|
|
name={"type" as keyof FormData}
|
|
value={data.type}
|
|
onChange={onChange}
|
|
/>
|
|
) : (
|
|
<>
|
|
<Typography className={classes.label} variant="caption">
|
|
<FormattedMessage defaultMessage="Attribute Class" />
|
|
</Typography>
|
|
<Typography>
|
|
{data.type === AttributeTypeEnum.PRODUCT_TYPE
|
|
? intl.formatMessage(messages.productAttribute)
|
|
: intl.formatMessage(messages.contentAttribute)}
|
|
</Typography>
|
|
</>
|
|
)}
|
|
</CardContent>
|
|
</Card>
|
|
);
|
|
};
|
|
AttributeOrganization.displayName = "AttributeOrganization";
|
|
export default AttributeOrganization;
|