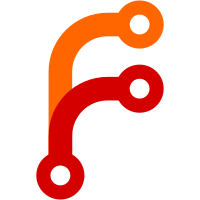
* create Apps view * create more app components, generate types and messages * apps refactor, update snapshots * show error message in tooltip when app installation fail * update apps components and view, add apps list to storybook * update defaultMessages * create app details view * update AppListPage with Skeleton component * create app activate/deactivate dialogs, create app details stories * add AppHeader to AppDetailsPage * update defaultMessages * update AppDetails view and components after review * create custom app details view * refactor webhooks * update webhooks fixtures * update WebhookDetailsPage story * update strings * create CustomAppCreate view and components * update AppListPage story * create AppInstall view and page * handle errors in AppInstall view * update defaultMessages * add AppInstallPage to storybook * add status prop to MessageManager * update defaultMessages * remove service account section * remove service account routes * remove as operator from notify status * add notifications for app installations * update styles for deactivated app * update app installations with local storage * update defaultMessages * AppInstall update * dd delete button to ongoin installations table * fix active installations condition * fix error messages in AppsList * update defaultMessages * add iframe to AppDetailsPage * create AppDetailsSettingsPage * install macaw-ui * apps styles clean up * update schema, fixtures * few apps updates * WebhookCreate - fix onBack button name * WebhookCreatePage story update * rename apps table from external to thirdparty * update defaultMessages * fix test, update snapshots * AppDetailsSettings - add token to headers * fix first number in local apps query * app details settings - use shop domain host * add onSettingsRowClick to InstalledApps * resolve conflicts * update changelog and messages * add noopener noreferrer do app privacy link * update snapshots * update snapshots * updates after review * update defaultMessages * CustomAppDetails - add missing notify status
121 lines
3.9 KiB
TypeScript
121 lines
3.9 KiB
TypeScript
import { AppErrorFragment } from "@saleor/apps/types/AppErrorFragment";
|
|
import AccountPermissions from "@saleor/components/AccountPermissions";
|
|
import AppHeader from "@saleor/components/AppHeader";
|
|
import AppStatus from "@saleor/components/AppStatus";
|
|
import CardSpacer from "@saleor/components/CardSpacer";
|
|
import { ConfirmButtonTransitionState } from "@saleor/components/ConfirmButton";
|
|
import Container from "@saleor/components/Container";
|
|
import Form from "@saleor/components/Form";
|
|
import Grid from "@saleor/components/Grid";
|
|
import PageHeader from "@saleor/components/PageHeader";
|
|
import SaveButtonBar from "@saleor/components/SaveButtonBar";
|
|
import { ShopInfo_shop_permissions } from "@saleor/components/Shop/types/ShopInfo";
|
|
import { sectionNames } from "@saleor/intl";
|
|
import { PermissionEnum } from "@saleor/types/globalTypes";
|
|
import { getFormErrors } from "@saleor/utils/errors";
|
|
import getAppErrorMessage from "@saleor/utils/errors/app";
|
|
import React from "react";
|
|
import { useIntl } from "react-intl";
|
|
|
|
import CustomAppInformation from "../CustomAppInformation";
|
|
|
|
export interface CustomAppCreatePageFormData {
|
|
hasFullAccess: boolean;
|
|
isActive: boolean;
|
|
name: string;
|
|
permissions: PermissionEnum[];
|
|
}
|
|
export interface CustomAppCreatePageProps {
|
|
disabled: boolean;
|
|
errors: AppErrorFragment[];
|
|
permissions: ShopInfo_shop_permissions[];
|
|
saveButtonBarState: ConfirmButtonTransitionState;
|
|
onBack: () => void;
|
|
onSubmit: (data: CustomAppCreatePageFormData) => void;
|
|
}
|
|
|
|
const CustomAppCreatePage: React.FC<CustomAppCreatePageProps> = props => {
|
|
const {
|
|
disabled,
|
|
errors,
|
|
permissions,
|
|
saveButtonBarState,
|
|
onBack,
|
|
onSubmit
|
|
} = props;
|
|
const intl = useIntl();
|
|
|
|
const initialForm: CustomAppCreatePageFormData = {
|
|
hasFullAccess: false,
|
|
isActive: false,
|
|
name: "",
|
|
permissions: []
|
|
};
|
|
|
|
const formErrors = getFormErrors(["permissions"], errors || []);
|
|
const permissionsError = getAppErrorMessage(formErrors.permissions, intl);
|
|
|
|
return (
|
|
<Form initial={initialForm} onSubmit={onSubmit} confirmLeave>
|
|
{({ data, change, hasChanged, submit }) => (
|
|
<Container>
|
|
<AppHeader onBack={onBack}>
|
|
{intl.formatMessage(sectionNames.apps)}
|
|
</AppHeader>
|
|
<PageHeader
|
|
title={intl.formatMessage({
|
|
defaultMessage: "Create New App",
|
|
description: "header"
|
|
})}
|
|
/>
|
|
<Grid>
|
|
<div>
|
|
<CustomAppInformation
|
|
data={data}
|
|
disabled={disabled}
|
|
errors={errors}
|
|
onChange={change}
|
|
/>
|
|
</div>
|
|
<AccountPermissions
|
|
data={data}
|
|
errorMessage={permissionsError}
|
|
disabled={disabled}
|
|
permissions={permissions}
|
|
permissionsExceeded={false}
|
|
onChange={change}
|
|
fullAccessLabel={intl.formatMessage({
|
|
defaultMessage: "Grant this app full access to the store",
|
|
description: "checkbox label"
|
|
})}
|
|
description={intl.formatMessage({
|
|
defaultMessage:
|
|
"Expand or restrict app permissions to access certain part of Saleor system.",
|
|
description: "card description"
|
|
})}
|
|
/>
|
|
<CardSpacer />
|
|
<AppStatus
|
|
data={data}
|
|
disabled={disabled}
|
|
label={intl.formatMessage({
|
|
defaultMessage: "App is active",
|
|
description: "checkbox label"
|
|
})}
|
|
onChange={change}
|
|
/>
|
|
</Grid>
|
|
<SaveButtonBar
|
|
disabled={disabled || !hasChanged}
|
|
state={saveButtonBarState}
|
|
onCancel={onBack}
|
|
onSave={submit}
|
|
/>
|
|
</Container>
|
|
)}
|
|
</Form>
|
|
);
|
|
};
|
|
|
|
CustomAppCreatePage.displayName = "CustomAppCreatePage";
|
|
export default CustomAppCreatePage;
|