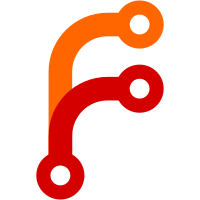
* Add allocation strategy options in channel view * Update schema with channel allocation strategy * Reorder channel warehouses after channel saving * Refactor and clean code of allocation strategy options * Update schema with allocation strategy * Trigger CI * Update allocation starategy options UI * Update allocation strategy messages * Trigger CI * Fix shipping zones and warehouses cards style * Fix message * Fix snapshots Co-authored-by: Michał Droń <dron.official@yahoo.com>
76 lines
1.5 KiB
TypeScript
76 lines
1.5 KiB
TypeScript
import { gql } from "@apollo/client";
|
|
|
|
export const channelCreateMutation = gql`
|
|
mutation ChannelCreate($input: ChannelCreateInput!) {
|
|
channelCreate(input: $input) {
|
|
channel {
|
|
...ChannelDetails
|
|
}
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const channelUpdateMutation = gql`
|
|
mutation ChannelUpdate($id: ID!, $input: ChannelUpdateInput!) {
|
|
channelUpdate(id: $id, input: $input) {
|
|
channel {
|
|
...ChannelDetails
|
|
}
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const channelDeleteMutation = gql`
|
|
mutation ChannelDelete($id: ID!, $input: ChannelDeleteInput) {
|
|
channelDelete(id: $id, input: $input) {
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const channelActivateMutation = gql`
|
|
mutation ChannelActivate($id: ID!) {
|
|
channelActivate(id: $id) {
|
|
channel {
|
|
...ChannelDetails
|
|
}
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const channelDeactivateMutation = gql`
|
|
mutation ChannelDeactivate($id: ID!) {
|
|
channelDeactivate(id: $id) {
|
|
channel {
|
|
...ChannelDetails
|
|
}
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|
|
|
|
export const ChannelReorderWarehousesMutation = gql`
|
|
mutation ChannelReorderWarehouses($channelId: ID!, $moves: [ReorderInput!]!) {
|
|
channelReorderWarehouses(channelId: $channelId, moves: $moves) {
|
|
channel {
|
|
...ChannelDetails
|
|
}
|
|
errors {
|
|
...ChannelError
|
|
}
|
|
}
|
|
}
|
|
`;
|