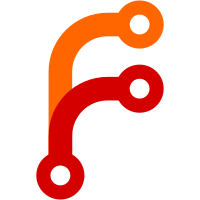
* feat: ✅ add first tests & use address-factory * feat: ✨ add distributeDiscount * refactor: taxjar maps to adapters (#495) * refactor: ♻️ taxjar-calculate-taxes-map with taxjar-calculate-taxes-adapter * refactor: ♻️ taxjar-order-created-map -> taxjar-order-created-adapter * refactor: ♻️ address 1st batch of feedback * refactor: ♻️ split up taxjar-calculate-taxes-adapter * refactor: 🚚 extract shipping transformer * docs: 💡 add comment about refunds in distribute-discount * refactor: 🚚 split up taxjar-order-created-adapter classes * refactor: ♻️ mocks with taxjar-mock-factory * refactor: ♻️ mocks with avatax-mock-factory * refactor: avatax maps to adapters (#506) * refactor: ♻️ move around & refactor avatax-order-created-map -> adapter * refactor: 🚚 move avatax-order-created- to its own folder * refactor: ♻️ avatax-calculate-taxes-map -> adapter * refactor: ♻️ avatax-order-fulfilled-maps -> adapter * feat: ✨ add logger to adapters * refactor: ♻️ mocks -> avatax-mock-transaction-factory & fix tests * feat: add tests for taxjar (#509) * fix: 🚚 tax-provider-utils.test name * feat: ✅ add nexus tests & other taxjar tests * feat: 🥅 add ExpectedError and use it in webhook-response * refactor: ✅ unify taxjar-calculate-taxes tests with mock-generator * feat: ✅ add TaxJarOrderCreatedMockGenerator * feat: ✅ add avatax-calculate-taxes-mock-generator * feat: ✅ add AvataxOrderCreatedMockGenerator * refactor: 🔥 tax-mock-factory * fix: 🐛 housekeeping * fix: 🐛 feedback * feat: ✅ add taxBase with discounts test * fix: 🐛 address feedback * refactor: 🔥 unused avatax-mock-factory functions * feat: ✨ use discount utils in all providers * feat: ✨ differentiate between pricesEnteredWithTax in taxjar
2301 lines
64 KiB
TypeScript
2301 lines
64 KiB
TypeScript
import { AdjustmentReason } from "avatax/lib/enums/AdjustmentReason";
|
|
import { BoundaryLevel } from "avatax/lib/enums/BoundaryLevel";
|
|
import { ChargedTo } from "avatax/lib/enums/ChargedTo";
|
|
import { DocumentStatus } from "avatax/lib/enums/DocumentStatus";
|
|
import { DocumentType } from "avatax/lib/enums/DocumentType";
|
|
import { JurisTypeId } from "avatax/lib/enums/JurisTypeId";
|
|
import { JurisdictionType } from "avatax/lib/enums/JurisdictionType";
|
|
import { LiabilityType } from "avatax/lib/enums/LiabilityType";
|
|
import { RateType } from "avatax/lib/enums/RateType";
|
|
import { TransactionModel } from "avatax/lib/models/TransactionModel";
|
|
|
|
const taxExcludedNoShipping: Partial<TransactionModel> = {
|
|
totalAmount: 80,
|
|
totalExempt: 0,
|
|
totalDiscount: 0,
|
|
totalTax: 7.6,
|
|
totalTaxable: 80,
|
|
totalTaxCalculated: 7.6,
|
|
lines: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "1",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 40,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date(new Date("2023-05-23")),
|
|
tax: 3.8,
|
|
taxableAmount: 40,
|
|
taxCalculated: 3.8,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date(new Date("2023-05-23")),
|
|
taxIncluded: false,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.4,
|
|
reportingTaxCalculated: 2.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.1,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.1,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.1,
|
|
reportingTaxCalculated: 0.1,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.9,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.9,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.9,
|
|
reportingTaxCalculated: 0.9,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.4,
|
|
reportingTaxCalculated: 0.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "2",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 40,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date(new Date("2023-05-23")),
|
|
tax: 3.8,
|
|
taxableAmount: 40,
|
|
taxCalculated: 3.8,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date(new Date("2023-05-23")),
|
|
taxIncluded: false,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.4,
|
|
reportingTaxCalculated: 2.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.1,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.1,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.1,
|
|
reportingTaxCalculated: 0.1,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.9,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.9,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.9,
|
|
reportingTaxCalculated: 0.9,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.4,
|
|
reportingTaxCalculated: 0.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
],
|
|
summary: [
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.State,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA STATE TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.06,
|
|
tax: 4.8,
|
|
taxCalculated: 4.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.County,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.0025,
|
|
tax: 0.2,
|
|
taxCalculated: 0.2,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "19",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.01,
|
|
tax: 0.8,
|
|
taxCalculated: 0.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "594",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.0225,
|
|
tax: 1.8,
|
|
taxCalculated: 1.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
],
|
|
};
|
|
|
|
const taxExcludedShipping: Partial<TransactionModel> = {
|
|
totalAmount: 157.51,
|
|
totalExempt: 0,
|
|
totalDiscount: 0,
|
|
totalTax: 14.96,
|
|
totalTaxable: 157.51,
|
|
totalTaxCalculated: 14.96,
|
|
lines: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "1",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 40,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.8,
|
|
taxableAmount: 40,
|
|
taxCalculated: 3.8,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: false,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.4,
|
|
reportingTaxCalculated: 2.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.1,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.1,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.1,
|
|
reportingTaxCalculated: 0.1,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.9,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.9,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.9,
|
|
reportingTaxCalculated: 0.9,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.4,
|
|
reportingTaxCalculated: 0.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "2",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 40,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.8,
|
|
taxableAmount: 40,
|
|
taxCalculated: 3.8,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: false,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.4,
|
|
reportingTaxCalculated: 2.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.1,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.1,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.1,
|
|
reportingTaxCalculated: 0.1,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.9,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.9,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.9,
|
|
reportingTaxCalculated: 0.9,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.4,
|
|
taxableAmount: 40,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.4,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 40,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.4,
|
|
reportingTaxCalculated: 0.4,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "3",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "Shipping",
|
|
lineAmount: 77.51,
|
|
quantity: 1,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 7.36,
|
|
taxableAmount: 77.51,
|
|
taxCalculated: 7.36,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: false,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 4.65,
|
|
taxableAmount: 77.51,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 4.65,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 77.51,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 4.65,
|
|
reportingTaxCalculated: 4.65,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.19,
|
|
taxableAmount: 77.51,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.19,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 77.51,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.19,
|
|
reportingTaxCalculated: 0.19,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 1.74,
|
|
taxableAmount: 77.51,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 1.74,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 77.51,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 1.74,
|
|
reportingTaxCalculated: 1.74,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.78,
|
|
taxableAmount: 77.51,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.78,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 77.51,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.78,
|
|
reportingTaxCalculated: 0.78,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
],
|
|
summary: [
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.State,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA STATE TAX",
|
|
rateType: RateType.General,
|
|
taxable: 157.51,
|
|
rate: 0.06,
|
|
tax: 9.45,
|
|
taxCalculated: 9.45,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.County,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
rateType: RateType.General,
|
|
taxable: 157.51,
|
|
rate: 0.0025,
|
|
tax: 0.39,
|
|
taxCalculated: 0.39,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "19",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 157.51,
|
|
rate: 0.01,
|
|
tax: 1.58,
|
|
taxCalculated: 1.58,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "594",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 157.51,
|
|
rate: 0.0225,
|
|
tax: 3.54,
|
|
taxCalculated: 3.54,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
],
|
|
};
|
|
|
|
const taxIncludedNoShipping: Partial<TransactionModel> = {
|
|
totalAmount: 73.06,
|
|
totalExempt: 0,
|
|
totalDiscount: 0,
|
|
totalTax: 6.94,
|
|
totalTaxable: 73.06,
|
|
totalTaxCalculated: 6.94,
|
|
lines: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "1",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 36.53,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.47,
|
|
taxableAmount: 36.53,
|
|
taxCalculated: 3.47,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.19,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.19,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.19,
|
|
reportingTaxCalculated: 2.19,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.09,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.09,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.09,
|
|
reportingTaxCalculated: 0.09,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.82,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.82,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.82,
|
|
reportingTaxCalculated: 0.82,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.37,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.37,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.37,
|
|
reportingTaxCalculated: 0.37,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "2",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 36.53,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.47,
|
|
taxableAmount: 36.53,
|
|
taxCalculated: 3.47,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.19,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.19,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.19,
|
|
reportingTaxCalculated: 2.19,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.09,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.09,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.09,
|
|
reportingTaxCalculated: 0.09,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.82,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.82,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.82,
|
|
reportingTaxCalculated: 0.82,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.37,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.37,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.37,
|
|
reportingTaxCalculated: 0.37,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
],
|
|
summary: [
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.State,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA STATE TAX",
|
|
rateType: RateType.General,
|
|
taxable: 73.06,
|
|
rate: 0.06,
|
|
tax: 4.38,
|
|
taxCalculated: 4.38,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.County,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
rateType: RateType.General,
|
|
taxable: 73.06,
|
|
rate: 0.0025,
|
|
tax: 0.18,
|
|
taxCalculated: 0.18,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "19",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 73.06,
|
|
rate: 0.01,
|
|
tax: 0.74,
|
|
taxCalculated: 0.74,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "594",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 73.06,
|
|
rate: 0.0225,
|
|
tax: 1.64,
|
|
taxCalculated: 1.64,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
],
|
|
};
|
|
|
|
const taxIncludedShipping: Partial<TransactionModel> = {
|
|
totalAmount: 143.84,
|
|
totalExempt: 0,
|
|
totalDiscount: 0,
|
|
totalTax: 13.67,
|
|
totalTaxable: 143.84,
|
|
totalTaxCalculated: 13.67,
|
|
lines: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "1",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 36.53,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.47,
|
|
taxableAmount: 36.53,
|
|
taxCalculated: 3.47,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.19,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.19,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.19,
|
|
reportingTaxCalculated: 2.19,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.09,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.09,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.09,
|
|
reportingTaxCalculated: 0.09,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.82,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.82,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.82,
|
|
reportingTaxCalculated: 0.82,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.37,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.37,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.37,
|
|
reportingTaxCalculated: 0.37,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "2",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "",
|
|
lineAmount: 36.53,
|
|
quantity: 2,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 3.47,
|
|
taxableAmount: 36.53,
|
|
taxCalculated: 3.47,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 2.19,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 2.19,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 2.19,
|
|
reportingTaxCalculated: 2.19,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.09,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.09,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.09,
|
|
reportingTaxCalculated: 0.09,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 0.82,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.82,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.82,
|
|
reportingTaxCalculated: 0.82,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.37,
|
|
taxableAmount: 36.53,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.37,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 36.53,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.37,
|
|
reportingTaxCalculated: 0.37,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "3",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 0,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: true,
|
|
itemCode: "Shipping",
|
|
lineAmount: 70.78,
|
|
quantity: 1,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date("2023-05-23"),
|
|
tax: 6.73,
|
|
taxableAmount: 70.78,
|
|
taxCalculated: 6.73,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date("2023-05-23"),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.06,
|
|
tax: 4.25,
|
|
taxableAmount: 70.78,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 4.25,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 70.78,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 4.25,
|
|
reportingTaxCalculated: 4.25,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.CTY,
|
|
jurisdictionType: JurisdictionType.County,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0025,
|
|
tax: 0.18,
|
|
taxableAmount: 70.78,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.18,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 70.78,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.18,
|
|
reportingTaxCalculated: 0.18,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
stateAssignedNo: "594",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.0225,
|
|
tax: 1.59,
|
|
taxableAmount: 70.78,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 1.59,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 70.78,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 1.59,
|
|
reportingTaxCalculated: 1.59,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "CA",
|
|
exemptAmount: 0,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
stateAssignedNo: "19",
|
|
jurisType: JurisTypeId.STJ,
|
|
jurisdictionType: JurisdictionType.Special,
|
|
nonTaxableAmount: 0,
|
|
rate: 0.01,
|
|
tax: 0.71,
|
|
taxableAmount: 70.78,
|
|
taxType: "Use",
|
|
taxSubTypeId: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0.71,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 70.78,
|
|
reportingNonTaxableUnits: 0,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0.71,
|
|
reportingTaxCalculated: 0.71,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
],
|
|
summary: [
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.State,
|
|
jurisCode: "06",
|
|
jurisName: "CALIFORNIA",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA STATE TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.06,
|
|
tax: 4.8,
|
|
taxCalculated: 4.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.County,
|
|
jurisCode: "037",
|
|
jurisName: "LOS ANGELES",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA COUNTY TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.0025,
|
|
tax: 0.2,
|
|
taxCalculated: 0.2,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMTC0",
|
|
jurisName: "LOS ANGELES CO LOCAL TAX SL",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "19",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.01,
|
|
tax: 0.8,
|
|
taxCalculated: 0.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
{
|
|
country: "US",
|
|
region: "CA",
|
|
jurisType: JurisdictionType.Special,
|
|
jurisCode: "EMAR0",
|
|
jurisName: "LOS ANGELES COUNTY DISTRICT TAX SP",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "594",
|
|
taxType: "Use",
|
|
taxSubType: "U",
|
|
taxName: "CA SPECIAL TAX",
|
|
rateType: RateType.General,
|
|
taxable: 80,
|
|
rate: 0.0225,
|
|
tax: 1.8,
|
|
taxCalculated: 1.8,
|
|
nonTaxable: 0,
|
|
exemption: 0,
|
|
},
|
|
],
|
|
};
|
|
|
|
const nonTaxable: Partial<TransactionModel> = {
|
|
totalAmount: 80,
|
|
totalExempt: 0,
|
|
totalDiscount: 0,
|
|
totalTax: 0,
|
|
totalTaxable: 0,
|
|
totalTaxCalculated: 0,
|
|
lines: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "1",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 20,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: false,
|
|
itemCode: "",
|
|
lineAmount: 20,
|
|
quantity: 1,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date(),
|
|
tax: 0,
|
|
taxableAmount: 0,
|
|
taxCalculated: 0,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date(),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "DE",
|
|
exemptAmount: 0,
|
|
jurisCode: "10",
|
|
jurisName: "DELAWARE",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 20,
|
|
rate: 0,
|
|
tax: 0,
|
|
taxableAmount: 0,
|
|
taxType: "Sales",
|
|
taxSubTypeId: "S",
|
|
taxName: "DE STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 0,
|
|
reportingNonTaxableUnits: 20,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0,
|
|
reportingTaxCalculated: 0,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
lineNumber: "2",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
discountAmount: 0,
|
|
exemptAmount: 77.51,
|
|
exemptCertId: 0,
|
|
exemptNo: "",
|
|
isItemTaxable: false,
|
|
itemCode: "Shipping",
|
|
lineAmount: 77.51,
|
|
quantity: 1,
|
|
ref1: "",
|
|
ref2: "",
|
|
reportingDate: new Date(),
|
|
tax: 0,
|
|
taxableAmount: 0,
|
|
taxCalculated: 0,
|
|
taxCode: "P0000000",
|
|
taxCodeId: 8087,
|
|
taxDate: new Date(),
|
|
taxIncluded: true,
|
|
details: [
|
|
{
|
|
id: 0,
|
|
transactionLineId: 0,
|
|
transactionId: 0,
|
|
country: "US",
|
|
region: "DE",
|
|
exemptAmount: 0,
|
|
jurisCode: "10",
|
|
jurisName: "DELAWARE",
|
|
stateAssignedNo: "",
|
|
jurisType: JurisTypeId.STA,
|
|
jurisdictionType: JurisdictionType.State,
|
|
nonTaxableAmount: 77.51,
|
|
rate: 0,
|
|
tax: 0,
|
|
taxableAmount: 0,
|
|
taxType: "Sales",
|
|
taxSubTypeId: "S",
|
|
taxName: "DE STATE TAX",
|
|
taxAuthorityTypeId: 45,
|
|
taxCalculated: 0,
|
|
rateType: RateType.General,
|
|
rateTypeCode: "G",
|
|
unitOfBasis: "PerCurrencyUnit",
|
|
isNonPassThru: false,
|
|
isFee: false,
|
|
reportingTaxableUnits: 0,
|
|
reportingNonTaxableUnits: 77.51,
|
|
reportingExemptUnits: 0,
|
|
reportingTax: 0,
|
|
reportingTaxCalculated: 0,
|
|
liabilityType: LiabilityType.Seller,
|
|
chargedTo: ChargedTo.Buyer,
|
|
},
|
|
],
|
|
nonPassthroughDetails: [],
|
|
hsCode: "",
|
|
costInsuranceFreight: 0,
|
|
vatCode: "",
|
|
vatNumberTypeId: 0,
|
|
},
|
|
],
|
|
summary: [
|
|
{
|
|
country: "US",
|
|
region: "DE",
|
|
jurisType: JurisdictionType.State,
|
|
jurisCode: "10",
|
|
jurisName: "DELAWARE",
|
|
taxAuthorityType: 45,
|
|
stateAssignedNo: "",
|
|
taxType: "Sales",
|
|
taxSubType: "S",
|
|
taxName: "DE STATE TAX",
|
|
rateType: RateType.General,
|
|
taxable: 0,
|
|
rate: 0,
|
|
tax: 0,
|
|
taxCalculated: 0,
|
|
nonTaxable: 80,
|
|
exemption: 0,
|
|
},
|
|
],
|
|
};
|
|
|
|
const defaultTransaction: TransactionModel = {
|
|
id: 0,
|
|
code: "fd334912-db04-4479-8e8b-c274c68ff4b5",
|
|
companyId: 7799660,
|
|
date: new Date(new Date("2023-05-23")),
|
|
paymentDate: new Date(new Date("2023-05-23")),
|
|
status: DocumentStatus.Temporary,
|
|
type: DocumentType.SalesOrder,
|
|
batchCode: "",
|
|
currencyCode: "USD",
|
|
exchangeRateCurrencyCode: "USD",
|
|
customerUsageType: "",
|
|
entityUseCode: "",
|
|
customerVendorCode: "VXNlcjoyMDg0NTEwNDEw",
|
|
customerCode: "VXNlcjoyMDg0NTEwNDEw",
|
|
exemptNo: "",
|
|
reconciled: false,
|
|
locationCode: "",
|
|
reportingLocationCode: "",
|
|
purchaseOrderNo: "",
|
|
referenceCode: "",
|
|
salespersonCode: "",
|
|
|
|
adjustmentReason: AdjustmentReason.NotAdjusted,
|
|
locked: false,
|
|
version: 1,
|
|
exchangeRateEffectiveDate: new Date(new Date("2023-05-23")),
|
|
exchangeRate: 1,
|
|
modifiedDate: new Date(new Date("2023-05-23T09:49:36.29194Z")),
|
|
modifiedUserId: 6479978,
|
|
taxDate: new Date(new Date("2023-05-23")),
|
|
lines: [],
|
|
addresses: [
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
boundaryLevel: BoundaryLevel.Zip5,
|
|
line1: "123 Palm Grove Ln",
|
|
line2: "",
|
|
line3: "",
|
|
city: "LOS ANGELES",
|
|
region: "CA",
|
|
postalCode: "90002",
|
|
country: "US",
|
|
taxRegionId: 4017056,
|
|
latitude: "33.948712",
|
|
longitude: "-118.245951",
|
|
},
|
|
{
|
|
id: 0,
|
|
transactionId: 0,
|
|
boundaryLevel: BoundaryLevel.Zip5,
|
|
line1: "8559 Lake Avenue",
|
|
line2: "",
|
|
line3: "",
|
|
city: "New York",
|
|
region: "NY",
|
|
postalCode: "10001",
|
|
country: "US",
|
|
taxRegionId: 2088629,
|
|
latitude: "40.748481",
|
|
longitude: "-73.993125",
|
|
},
|
|
],
|
|
summary: [],
|
|
};
|
|
|
|
const mockTransactionVariants = {
|
|
taxExcludedNoShipping,
|
|
taxExcludedShipping,
|
|
taxIncludedNoShipping,
|
|
taxIncludedShipping,
|
|
nonTaxable,
|
|
};
|
|
|
|
type MockTransactionVariant = keyof typeof mockTransactionVariants;
|
|
|
|
const createMockTransaction = (
|
|
variant: MockTransactionVariant,
|
|
overrides?: Partial<TransactionModel>
|
|
): TransactionModel => ({
|
|
...defaultTransaction,
|
|
...mockTransactionVariants[variant],
|
|
...overrides,
|
|
});
|
|
|
|
// todo: convert to a mock-generator
|
|
export const avataxMockTransactionFactory = {
|
|
createMockTransaction,
|
|
};
|