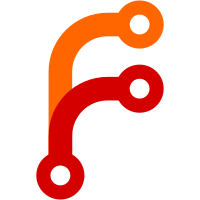
* WIP * Added script and making implementation more roboust * Added rollback on issues with the migration * Cleanup the code * Use allSettled instead of all * Do not check spelling in schema files. Schema is pulled from the API and is not controlled by our team * Update the pkg json * Fix typo on log message * Add dedupe to the ignored words. Its used by codegen * Add changesets
26 lines
835 B
TypeScript
26 lines
835 B
TypeScript
import { Client } from "urql";
|
|
import { WebhookDetailsFragment } from "../generated/graphql";
|
|
import { modifyAppWebhook } from "./operations/modify-app-webhook";
|
|
|
|
interface ModifyAppWebhookFromWebhookDetailsArgs {
|
|
client: Client;
|
|
webhookDetails: WebhookDetailsFragment;
|
|
}
|
|
|
|
export const modifyAppWebhookFromWebhookDetails = async ({
|
|
client,
|
|
webhookDetails,
|
|
}: ModifyAppWebhookFromWebhookDetailsArgs) => {
|
|
return modifyAppWebhook({
|
|
client,
|
|
webhookId: webhookDetails.id,
|
|
input: {
|
|
asyncEvents: webhookDetails.asyncEvents.map((event) => event.eventType),
|
|
syncEvents: webhookDetails.syncEvents.map((event) => event.eventType),
|
|
isActive: webhookDetails.isActive,
|
|
name: webhookDetails.name,
|
|
targetUrl: webhookDetails.targetUrl,
|
|
query: webhookDetails.subscriptionQuery,
|
|
},
|
|
});
|
|
};
|