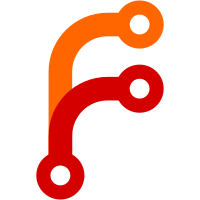
Add debug messages to middlewares Apply suggestions from code review Co-authored-by: Krzysztof Wolski <krzysztof.k.wolski@gmail.com> better debug messages Add experimental debug middleware
27 lines
885 B
TypeScript
27 lines
885 B
TypeScript
import { Handler, Request } from "retes";
|
|
import { Response } from "retes/response";
|
|
import { describe, expect, it, vi } from "vitest";
|
|
|
|
import { withReqResDebugging } from "./middleware-debug";
|
|
|
|
describe("withReqResDebugging", () => {
|
|
it("Logs request and response to debug", async () => {
|
|
const mockDebug = vi.fn();
|
|
const handler: Handler = async () => Response.OK("Tested handler is ok");
|
|
const wrappedHandler = withReqResDebugging(() => mockDebug)(handler);
|
|
|
|
const mockReqBody = JSON.stringify({ foo: "bar" });
|
|
|
|
await wrappedHandler({ rawBody: mockReqBody } as Request);
|
|
|
|
expect(mockDebug).toHaveBeenNthCalledWith(1, "Called with request %j", {
|
|
rawBody: mockReqBody,
|
|
});
|
|
|
|
expect(mockDebug).toHaveBeenNthCalledWith(2, "Responded with response %j", {
|
|
body: "Tested handler is ok",
|
|
headers: {},
|
|
status: 200,
|
|
});
|
|
});
|
|
});
|